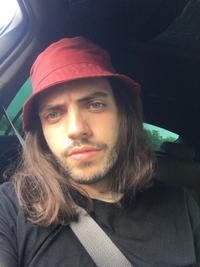
Sergen Demir
2022-11-10
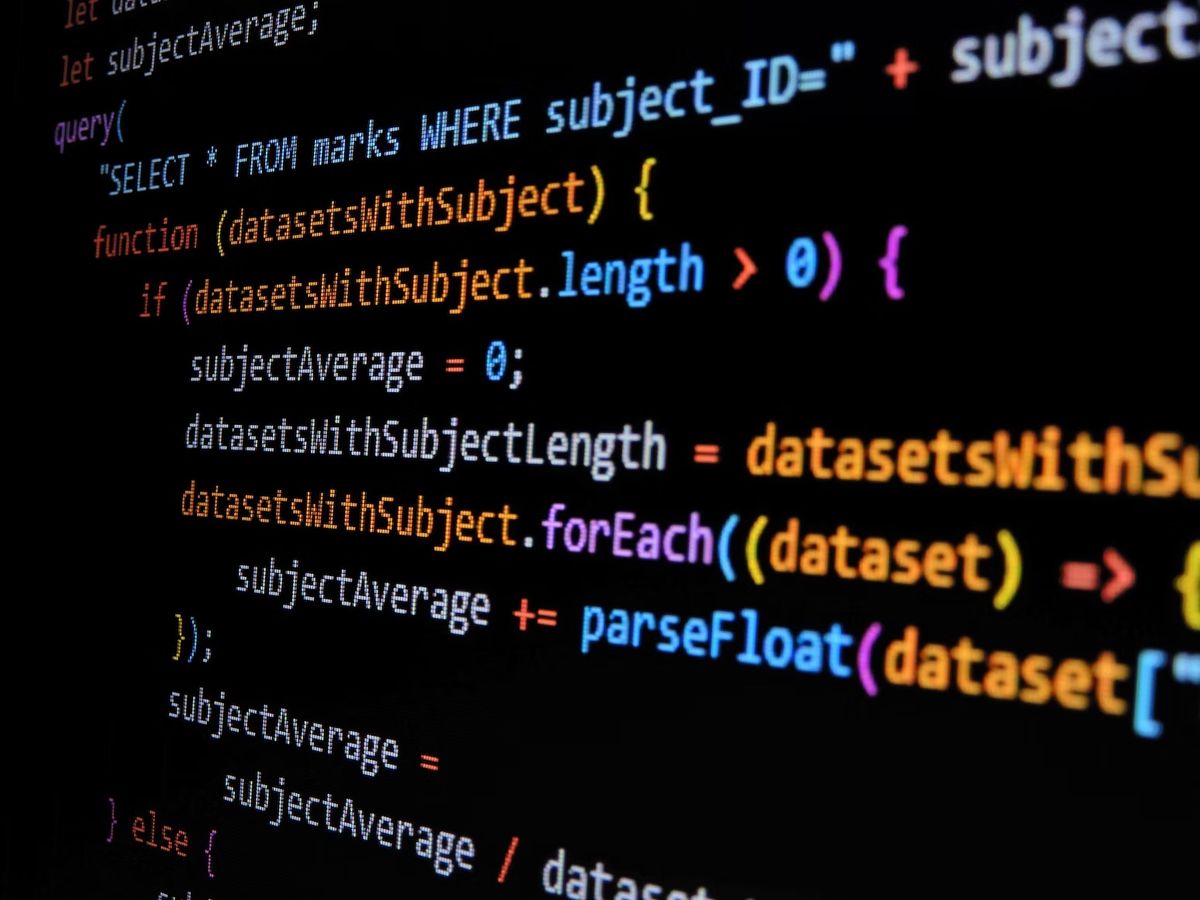
DOM Manipulation for Beginners
Methods to Manipulate the DOM Using JavaScript
JavaScript is one of the most popular programming languages out there. The possibilities of what you can build with it are limitless. The community behind it is great, anyone can easily find help if they need it.
For beginners some concepts could be a little bit more challenging to understand than others. Especially after learning HTML and CSS and moving onto Javascript can be very daunting, because it's nothing like you have encountered before. At that moment, some beginners might want to jump to a JS Framework instead of learning Vanilla JS, which I would not suggest. While JS Frameworks like React.js, Vue.js and Angular.js can make things easier, it's better to learn JavaScript fundamentals first.
Why do we need JS if we can create static websites only with HTML and CSS?
Javascript is the engine of your website/ web app. You can dynamically update/add/delete elements, add interactivity to your website and improve user experience.
I know this from experience, for a beginner to continue learning JS, they need small wins every day. At least, that's how I kept going, we need to see that we can actually create things on a web page using JS. That's where the DOM Manipulation becomes handy. Because you can create something as simple as dark - light mode switch, and it could be a small win in your journey.
What is the DOM?
The Document Object Model (DOM) is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content. The DOM represents the document as nodes and objects; that way, programming languages can interact with the page (MDN Web Docs).
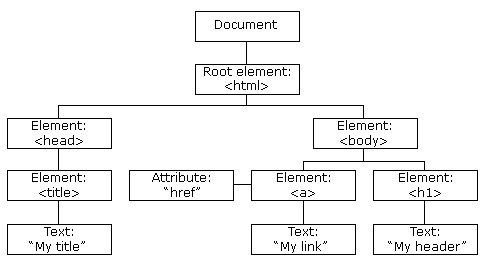
DOM manipulation was definitely one of the challenging things to grasp for me when I was starting out with JavaScript. But I had a breakthrough with JavaScript30 course by Wes Bos. While the course is not solely focused on DOM manipulation you can learn JS fundamentals in just 30 days. I learned about built-in javascript functions, window object methods, array methods etc. I would suggest everyone who is learning JavaScript to check out the course, and it's FREE!
- Here is the link to the course : https://javascript30.com/
Key Methods For Beginners
There are a few key methods that beginners can start using today that would get them motivated to keep learning. It's actually much simpler than you think. Every element in the DOM can be accessed using one or more of the methods shown below. You can decide how you want to access the element. You can access elements by class name, id, tag name etc...
- querySelector
- getElementById
- getElementByClassName
- getElementsByTagName
Accessing Elements from the DOM
```
// index.html
<body>
<h1 class="title" id="title">Hello World!</h1>
<button class="button" id="button">Change Title</button>
<div class="container"></div>
</body>
// app.js
const title = document.querySelector('.title');
const button = document.querySelector('.button');
const container = document.querySelector('.container');
// or
const title = document.getElementById('title');
const button = document.getElementById('button');
// or
const title = document.getElementsByTagName('h1'); // this will return all the h1 elements in the DOM.
```
Once you have access to the elements you want to target, you can use methods shown below to dynamically manipulate those elements.
- element.addEventListener(event, function) - event: 'click', 'mouseover' and many more
- element.createElement(element) - element: 'div', 'p', 'h1' etc...
- element.getAttribute('id') - getting attribute of an element (id, class, etc.)
- element.setAttribute('attribute', 'value') - setting attribute to an element (id, class etc.)
- element.appendChild(element) - single element
- element.append(element1, element2) - multiple elements
- element.classList - .add, .remove , .toggle
- element.innerText
- element.innerHTML
```
// app.js
const title = document.querySelector(".title");
const button = document.querySelector(".button");
const container = document.querySelector(".container");
function createCircle() {
const circle = document.createElement("div");
circle.classList.add("circle"); // adding a class name to newly created element.
container.appendChild(circle); // append newly created element to the container element in the DOM.
}
button.addEventListener("click", () => {
title.innerText = "Title has been changed! A new Circle has been added!";
createCircle();
});
// styles.css
.circle {
width: 50px;
height: 50px;
border-radius: 50%;
background-color: red;
}
```
- I suggest https://www.w3schools.com/ and https://developer.mozilla.org/en-US/ to learn so much more... Take your time and focus on one thing at a time!
Conclusion
As you can see learning JavaScript does not have to be a dark scary journey. You just need a structure and consistency. you need to trust the process! Getting comfortable with the DOM and then learning array methods and data structures will set you for success in the future.
I hope this short blog provided some value in any way, shape, or form. And don't forget, you got this!
Please feel free to correct me if I made a mistake explaining a concept or a method. Also feel free to contact me if you have any questions and let's connect on social media!